Python in Action: A Project-Based Introduction to Python Programming
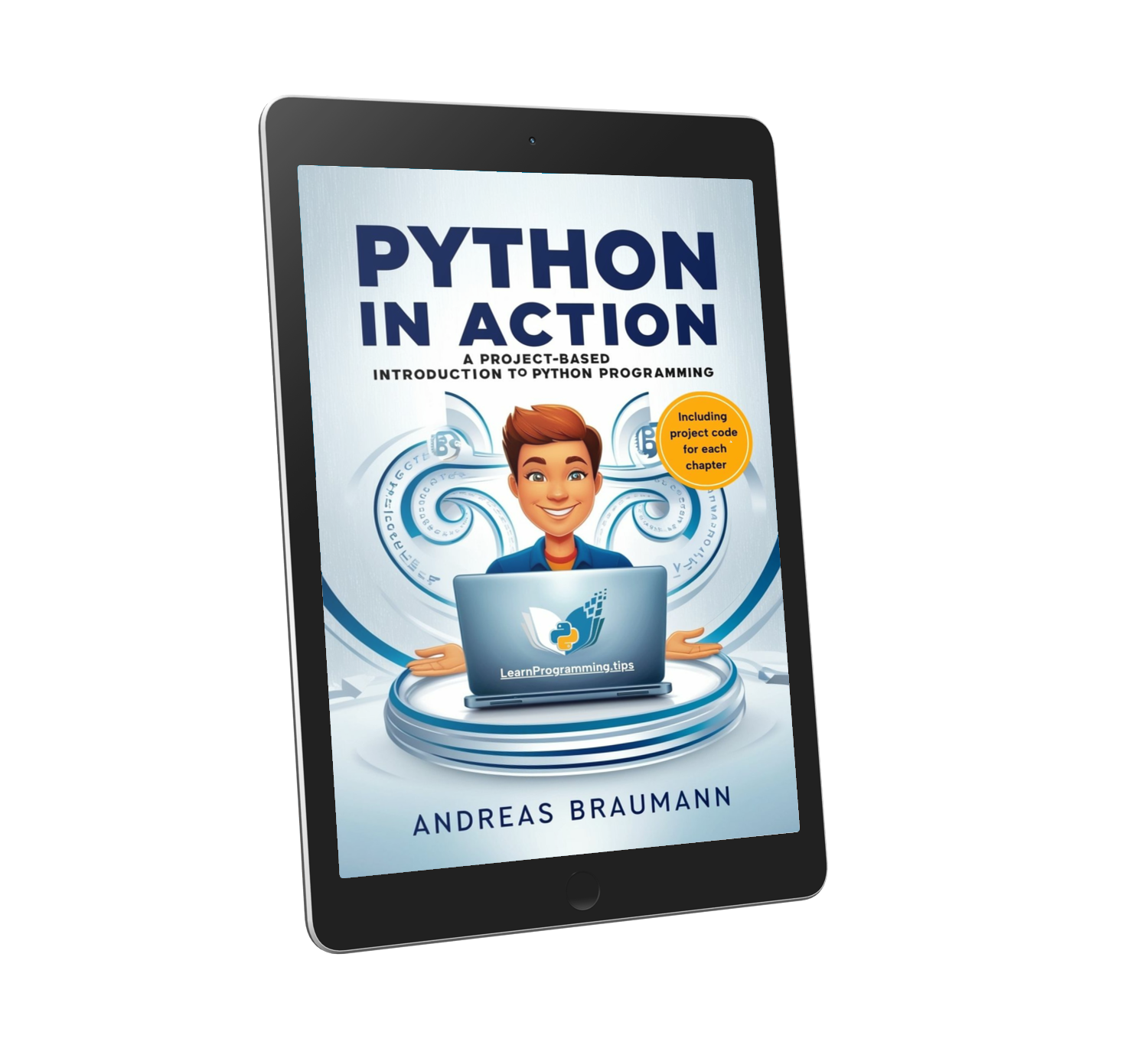
Master Python Through Real-World Projects
Ready to build real Python applications? This book teaches you Python with a hands-on, project-based approach — guiding you through real-world projects that apply everything you learn.
From building robust scripts to integrating with web services, you’ll gain the skills to tackle real development challenges.
By the end, you’ll be equipped to create, analyze, and deploy complete Python applications. Start mastering Python today!
Check out the code examples on our GitHub repository.
Andreas Braumann
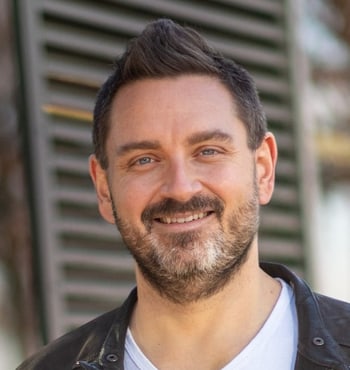
The author is a seasoned content creator and expert in AI and ChatGPT technologies. With a background as a Canva Engineering alumnus, he combines his technical acumen with creative flair to produce engaging, innovative content. Andreas is passionate about leveraging AI to enhance digital experiences and drive impactful storytelling.
Book Outline
Introduction
- Welcome to Python in Action
- Why Python?
- What You'll Learn in This Book
- How to Use This Book
- The Official GitHub Repository
- Recommended Resources
Chapter 1: Getting Started with Python
- Installing Python
- Setting Up Your Development Environment
- Writing Your First Python Script
- Understanding Python Syntax
- Basic Input and Output
Chapter 2: Variables, Data Types, and Operators
- Working with Variables
- Python Data Types
- Arithmetic, Comparison, and Logical Operators
- Basic String Manipulation
- Project: Simple Calculator
Chapter 3: Control Flow in Python
- Conditional Statements: if, elif, else
- Loops: for and while
- Breaking Out of Loops
- Working with Boolean Logic
- Project: Number Guessing Game
Chapter 4: Functions and Modules
- Defining and Calling Functions
- Function Parameters and Return Values
- Understanding Scope
- Using Modules and Packages
- Project: Basic To-Do List
Chapter 5: Working with Data Structures
- Lists, Tuples, and Sets
- Dictionaries and Key-Value Pairs
- List and Dictionary Comprehensions
- Project: Contact Book
Chapter 6: Object-Oriented Programming (OOP)
- Classes and Objects
- Attributes and Methods
- Inheritance and Polymorphism
- Encapsulation and Abstraction
- Project: Library Management System
Chapter 7: Handling Errors and Exceptions
- Understanding Exceptions
- Try, Except, and Finally Blocks
- Raising and Handling Custom Exceptions
- Project: Basic Banking Application
Chapter 8: File Handling
- Reading from Files
- Writing to Files
- Working with CSV and JSON Files
- Project: Student Grade Tracker
Chapter 9: Working with External Libraries
- Using pip to Install External Libraries
- Popular Libraries: requests, NumPy, Pandas
- Organizing Code into Modules
- Project: Weather App
Chapter 10: Advanced File Handling
- Working with Binary Files
- Buffered and Unbuffered File Operations
- Using Context Managers
- Project: File Backup Utility
Chapter 11: GUI Programming with Tkinter
- Introduction to Tkinter
- Creating Windows and Widgets
- Handling Events (Button Clicks, Input Validation)
- Laying Out a GUI Application
- Project: Desktop Weather Application
Chapter 12: Regular Expressions in Python
- Introduction to Regular Expressions
- Using Python's re Module
- Common Regex Patterns and Syntax
- Capturing Groups and Lookaheads
- Project: Text Pattern Finder
Chapter 13: Capstone Project – Build a Simple Game
- Recap of Major Concepts: Control Flow, Functions, OOP
- Introduction to Game Programming with Pygame
- Project: Simple Quiz Game
Chapter 14: Where to Go from Here – Expanding Your Python Knowledge
- Recommended Libraries and Frameworks
- Online Resources and Communities
- Best Practices for Writing Clean and Efficient Code
- Introduction to Version Control with Git and GitHub
Appendices
- Python Reference Sheet: Common Functions and Syntax
- Setting Up Python for Advanced Projects: Virtual Environments and Package Management
Read the Introduction and First Chapter
The preview is only available for signed-in users.
Sign Up